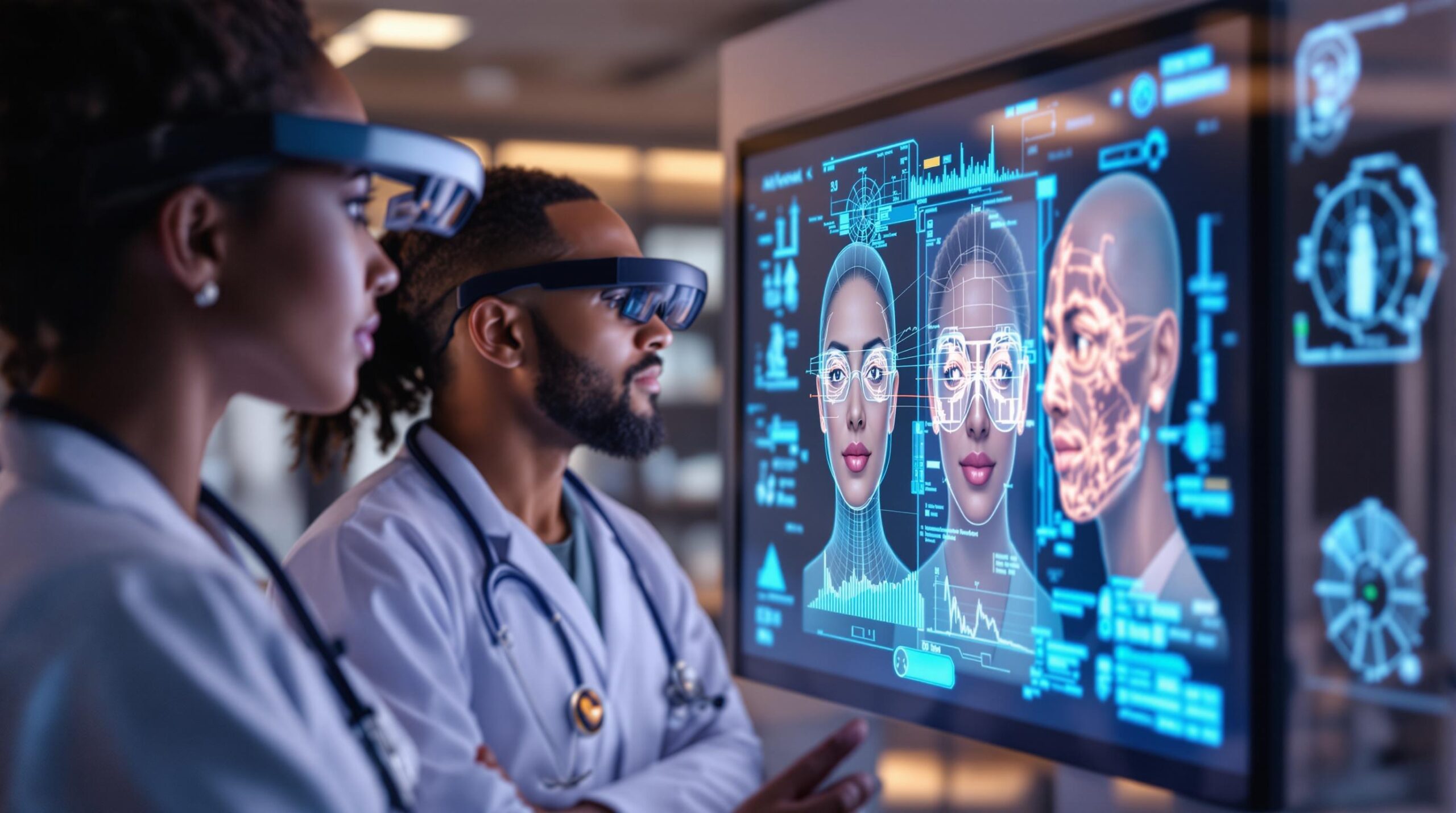
Apple’s ARKit uses the TrueDepth camera and machine learning to track 52 facial movements in real-time at 60fps. It creates a 3D face mesh with 1,220 points, detects expressions like blinking or smiling, and adapts to lighting changes. This technology powers healthcare tools like FaceStroke, which improves stroke assessments by 28%, and FacePalsy, which reduces facial paralysis evaluation time to 5 minutes. ARKit processes all data locally, ensuring privacy and HIPAA compliance.
Key Features:
- 3D Face Mesh: Tracks 1,220 points with real-time updates.
- Expression Detection: Monitors 52 facial muscle movements.
- Light Adaptation: Adjusts to ambient light for seamless AR experiences.
- Healthcare Applications: Used for stroke recovery, facial paralysis, and accessibility tools.
ARKit makes it easy for developers to create secure, medical-grade tools with accurate facial tracking. Below, learn how to set up ARKit face tracking, process real-time data, and ensure security.
How To Make Real Time ARKit 3D Face Tracker
ARKit Face Tracking Features
ARKit’s facial tracking system uses the TrueDepth camera to provide accurate and responsive facial analysis. By combining advanced hardware and software, it enables engaging AR experiences through three main features.
3D Face Mesh Generation
This feature creates a 3D mesh with 1,220 points that map out the structure and shape of a face[3]. The mesh updates in real-time at 60fps, even during facial movements. Thanks to infrared sensors, ARKit maintains its accuracy in different lighting conditions[1]. Its machine learning algorithms can handle situations where only part of the face is visible and can quickly resume full tracking when the face is fully visible again[3].
Facial Expression Detection
ARKit tracks 52 specific facial muscle movements using blend shapes that measure actions like:
Facial Region | Tracked Actions |
---|---|
Eyes | Blinking, gaze direction |
Mouth | Smiling, frowning, lip puckering |
Eyebrows | Raising, furrowing |
Cheeks | Puffing, muscle activity |
Nose | Wrinkling, nostril flaring |
Each blend shape value ranges from 0 to 1[1], allowing for detailed tracking of expression intensity. This precision is especially useful for medical applications like monitoring rehabilitation progress or creating highly detailed facial animations.
Light Detection System
ARKit’s light estimation feature evaluates ambient light, including its intensity, direction, and color temperature, to seamlessly blend virtual objects with real environments. Using the device’s camera, it adapts to changes in lighting conditions[1]. All processing is done locally on the device[4], ensuring user data stays private – an important factor for healthcare and research applications[1].
These features make ARKit an excellent choice for developing HIPAA-compliant medical tools, as described in later sections.
ARKit Face Tracking Setup Guide
Setting Up Face Tracking
To use ARKit’s face tracking features, start by ensuring your hardware is compatible. You’ll need a device with a TrueDepth camera. Also, add camera permissions to your app’s Info.plist
file:
<key>NSCameraUsageDescription</key>
<string>This app requires camera access for facial tracking.</string>
Next, set up face tracking with the following code:
import ARKit
class ViewController: UIViewController {
@IBOutlet weak var sceneView: ARSCNView!
override func viewDidLoad() {
super.viewDidLoad()
guard ARFaceTrackingConfiguration.isSupported else {
let alert = UIAlertController(title: "Device Unsupported",
message: "Face tracking requires TrueDepth camera.",
preferredStyle: .alert)
present(alert, animated: true)
return
}
let configuration = ARFaceTrackingConfiguration()
configuration.maximumNumberOfTrackedFaces = 1
sceneView.session.run(configuration)
}
}
Processing Facial Data
Processing facial data in real-time is important for applications like healthcare monitoring, where immediate feedback can be crucial. Use the following code to handle updates from ARKit:
func session(_ session: ARSession, didUpdate anchors: [ARAnchor]) {
guard let faceAnchor = anchors.first as? ARFaceAnchor else { return }
// Access common expressions
let expressions = [
"Smile": faceAnchor.blendShapes[.mouthSmileLeft],
"Eye Blink": faceAnchor.blendShapes[.eyeBlinkLeft],
"Eyebrow Raise": faceAnchor.blendShapes[.browInnerUp]
]
// Offload processing to maintain smooth tracking
DispatchQueue.global(qos: .userInitiated).async {
self.processExpressions(expressions)
}
}
This approach ensures the app maintains a smooth 60fps experience while processing facial data.
3D Face Visualization
To create a visual representation of the tracked face, use SceneKit. Here’s an example:
class FaceTrackingViewController: UIViewController {
private var faceNode: SCNNode?
func setupFaceTracking() {
guard let device = sceneView.device else { return }
let faceGeometry = ARSCNFaceGeometry(device: device)
faceNode = SCNNode(geometry: faceGeometry)
// Apply semi-transparent material for clear mesh visualization
let material = SCNMaterial()
material.diffuse.contents = UIColor.white.withAlphaComponent(0.7)
faceGeometry?.firstMaterial = material
sceneView.scene.rootNode.addChildNode(faceNode!)
}
}
The generated mesh can be used for medical tools, such as those analyzing facial symmetry in stroke recovery.
Feature | Specification | Impact |
---|---|---|
Processing Priority | User Initiated QoS | Keeps tracking responsive |
These steps provide the groundwork for the medical tracking applications that follow.
sbb-itb-7af2948
Medical and Research Uses
ARKit’s advanced features are opening up new possibilities in healthcare and research. Developers are leveraging its precision to create tools that benefit both medical professionals and patients in meaningful ways.
Medical Recovery Tracking
The FacePalsy app is a prime example of ARKit’s impact. It has cut evaluation times for conditions like Bell’s palsy and stroke-related facial paralysis from 30 minutes to just 5, all while maintaining 95% clinical accuracy [5]. This allows healthcare providers to monitor recovery progress more efficiently and gather valuable data for research.
Accessibility Tools
ARKit also supports innovative accessibility solutions. Eye-tracking interfaces and facial gesture controls are making technology more accessible for individuals with mobility challenges, offering new ways to interact with devices.
Research Data Collection
In research, ARKit’s precision is proving invaluable. Stanford University used a custom iOS app powered by ARKit to analyze facial expressions from 1,000 participants as part of an emotion recognition study [1].
"ARKit’s micro-expression detection enables new research possibilities previously unattainable through observation alone", says Dr. Chen from Stanford University’s medical research team [1].
To ensure these tools are both effective and secure, developers need to focus on strong encryption and local data processing. This approach allows for accurate patient monitoring while safeguarding privacy [2].
Technical Guidelines and Security
These technical steps are crucial for ensuring that medical tracking apps operate reliably and comply with regulatory standards. They help maintain clinical-grade accuracy while safeguarding sensitive biometric data.
Performance Tips
ARKit face tracking processes up to 52 facial muscle movements at 60 frames per second, which demands substantial computational power [1]. To keep things running smoothly, developers should prioritize a few key areas:
Device-Specific Adjustments
- Tailor rendering based on the device’s capabilities.
- Use lower-resolution meshes when high detail isn’t necessary.
- Keep an eye on frame rates and adjust processing loads as needed.
Efficient Resource Management
- Shift heavy computations to background threads.
- Pause sessions when face tracking is not in use.
- Cache frequently accessed assets to minimize runtime delays.
To address tracking inconsistencies, include quality monitoring systems. Using Metal for GPU acceleration can help manage complex facial data processing while ensuring stable performance.
Performance Factor | Approach |
---|---|
Frame Rate | Use Metal for GPU-accelerated rendering |
Memory Usage | Free up unused resources promptly |
Battery Life | Pause tracking during inactivity |
Data Security Protocol
ARKit processes data locally [4], which provides a solid foundation for privacy. Developers should take these additional steps to secure biometric data:
Key Security Practices:
- Apply AES-256 encryption for any stored facial data.
- Ensure all biometric data is processed locally, avoiding external servers.
- Use HTTPS/TLS protocols for any necessary data transmission.
These measures support compliance with standards like HIPAA, which is essential for tools such as FacePalsy. Clear, user-friendly consent mechanisms should also be in place to explain how data will be used.
Next Steps with ARKit
Once you’ve set up the core tracking features and security protocols, it’s time to dive into more advanced strategies to refine your implementation.
Development Best Practices
To improve tracking performance, consider these tips:
- Use dynamic feature scaling during periods of high CPU usage.
- Leverage Metal for GPU-accelerated rendering to boost visual performance.
- Focus on error handling to manage tracking failures effectively.
Integration with Apple Frameworks
Take your app to the next level by combining ARKit with tools like Core ML. This integration can enhance facial feature detection accuracy and enable deeper analysis of expressions, which is especially useful for medical applications that need precise measurements of facial movements [5].
Testing and Validation
If your project involves research or requires long-term data collection, thorough testing is key. Focus on:
- Performance monitoring: Measure CPU usage and frame rates across a variety of devices.
- Edge case testing: Experiment with different facial orientations and tracking limits.
- User interaction validation: Test with diverse user groups to ensure accurate results.
Preparing for Future Updates
Stay ahead by keeping an eye on ARKit updates through WWDC sessions. Updates often bring improved emotion detection and expanded facial feature tracking. Design your app architecture to support these new features while maintaining compatibility with current devices.
For medical tools that need to comply with HIPAA, make sure your app includes clear mechanisms for obtaining user consent when collecting and analyzing data [2].
FAQs
How does ARKit face tracking work?
ARKit’s face tracking uses the TrueDepth camera to create a detailed 3D map of a face. It captures facial features with a 1,220-point mesh and tracks 52 facial movements, all at 60 frames per second. By combining infrared imaging and machine learning, it delivers highly accurate facial analysis.
When face tracking is enabled, ARKit creates ARFaceAnchor objects that provide:
- The face’s position and orientation in 3D space
- Real-time data on facial expressions
- Blend shape coefficients for specific muscle movements
To use ARKit face tracking, you’ll need:
- An iOS device with a TrueDepth camera (iPhone X or newer)
- Access to the front-facing camera
This technology processes facial data directly on the device, making it ideal for creating interactive AR experiences like lifelike avatars, emotion recognition tools, and accessibility features. It also supports privacy-focused applications in healthcare, such as those used for recovery tracking.