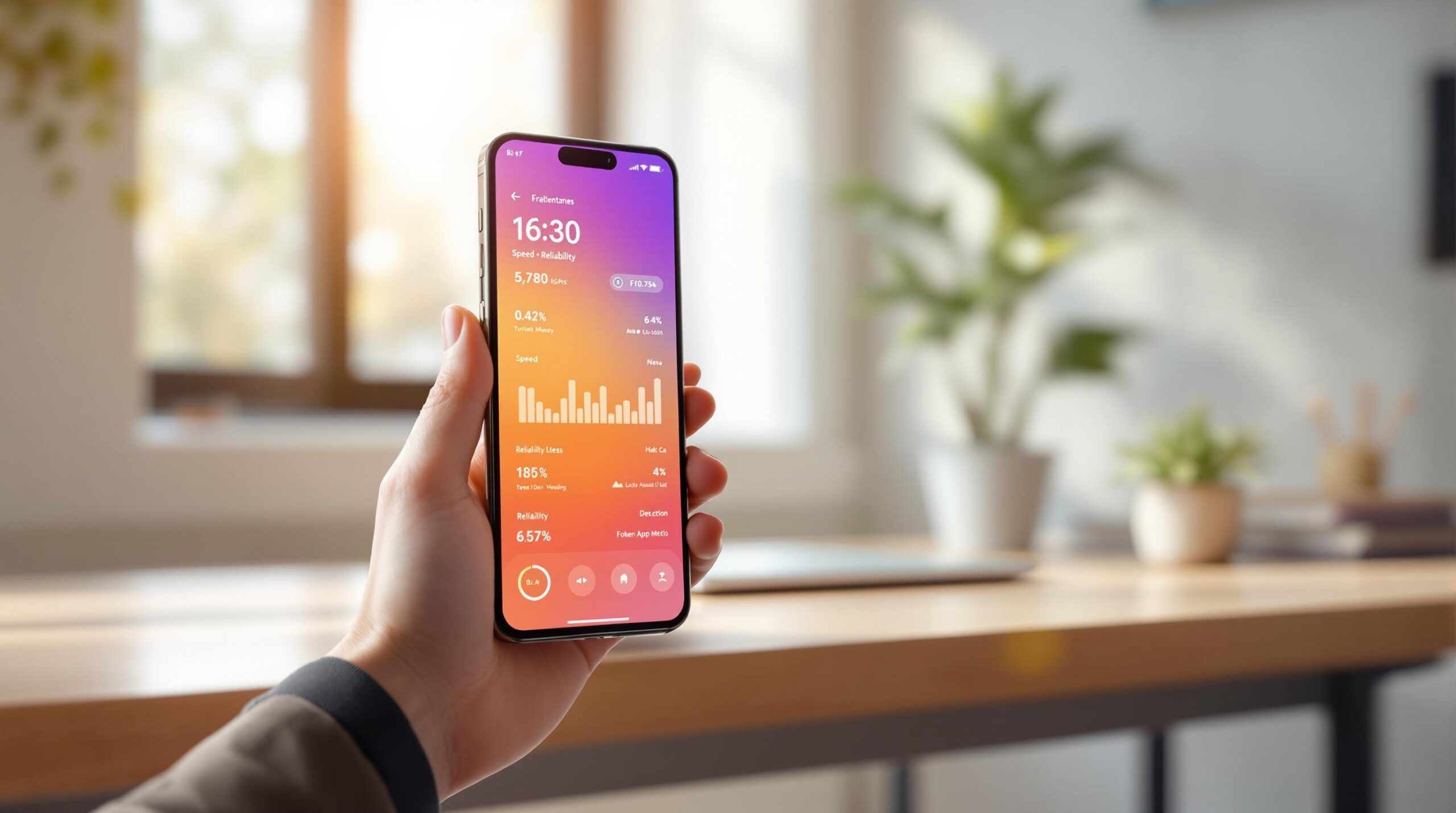
To create high-performing mobile apps, you need to focus on speed, reliability, and scalability. Users demand apps that load in under 3 seconds, and businesses that meet these expectations, like Airbnb, see real benefits – Airbnb boosted bookings by 10% after improving app speed. Here’s a quick rundown of what works:
- App Architecture: Use patterns like MVVM or modular design to ensure scalability and better performance.
- Efficient Code: Write clean, optimized code, leverage asynchronous programming, and manage memory smartly.
- Caching & Data Handling: Use local caching, CDNs, and optimized database queries to reduce load times.
- Network Optimization: Minimize API calls, compress data, and handle network issues with retries and better error management.
- Performance Monitoring: Use tools like Firebase or Xcode Instruments to test, debug, and track app performance.
These strategies can cut load times, prevent crashes, and improve user experience dramatically. Ready to dive deeper? Let’s break it all down step by step.
Optimizing Your Mobile App: Top Performance Tips
Designing App Architecture for Speed and Scalability
The architecture of a mobile app plays a critical role in its performance and growth potential. Making thoughtful decisions during the development phase can have a lasting impact on how well the app performs and adapts over time.
Choosing the Right Architecture
The architecture pattern you choose directly influences how well your app performs, how easily it scales, and how manageable the codebase remains. Modern patterns emphasize separating concerns and keeping the code clean and maintainable.
Architecture Pattern | Key Advantages |
---|---|
MVVM | Separates data and UI clearly, simplifies testing complex UI logic |
Clean Architecture | Layer independence, easier to manage large-scale apps |
MVC | Easy to implement, suitable for simpler apps |
Google’s Android team showcased how proper architecture can improve app reliability by up to 40% when using their Architecture Components libraries [1]. These tools help developers create apps with fewer crashes and better performance.
Leveraging Native Capabilities
Using platform-specific tools and languages like Swift and Kotlin can give your app a performance edge. These native options allow developers to tap into hardware directly, manage memory efficiently, and use platform-specific optimizations.
"Using Swift’s built-in parallel processing features has allowed us to improve app responsiveness by 30% while reducing memory usage by 25%" [2].
By fully utilizing native capabilities, you can build apps that are not only faster but also more reliable and responsive.
Building for Scalability with Modular Design
A modular approach is key to developing apps that can expand without losing efficiency. Breaking the app into smaller, independent modules ensures better resource allocation and simplifies updates or maintenance.
Instagram’s engineering team adopted a modular architecture, cutting their app’s initial load time in half by loading modules independently [4]. Their strategy included:
Module Type | Performance Benefit |
---|---|
Core Features | Quicker initial load |
Optional Features | Lower memory consumption |
Shared Resources | Efficient resource usage |
To replicate this, focus on creating self-contained modules for specific app functions. These modules can be developed and deployed separately, allowing you to add new features without disrupting existing ones.
Once your architecture is solid, the next step is ensuring your code aligns with these principles to maximize efficiency and reliability.
Writing Efficient Code for Reliable Apps
Efficient code is critical for ensuring your app runs fast, stays stable, and uses resources wisely. With a strong architecture in place, writing optimized code helps your app perform consistently well.
Writing Clean and Optimized Code
Modern coding practices emphasize clarity, maintainability, and performance.
Technique | How It Helps |
---|---|
Code Refactoring | Simplifies code structure and makes it easier to manage |
Using Native Code | Taps into device-specific features for better performance |
Memory Optimization | Avoids crashes and lagging issues |
Using Asynchronous Programming
Asynchronous programming keeps your app responsive by handling time-consuming tasks in the background.
"Asynchronous programming is a key concept in mobile development that enables time-consuming processes to be completed in the background, keeping applications responsive and overall performance high" [2].
Here’s how you can implement it:
Task | Async Approach |
---|---|
Network Requests | Use coroutines or promises |
Image Loading | Apply lazy loading techniques |
Database Operations | Run tasks on background threads |
Heavy Computations | Use worker threads for processing |
Managing Memory and Resources Smartly
Tools like Android Studio‘s Memory Monitor and LeakCanary can help you spot memory leaks early, preventing performance issues [3].
Tips for better memory management:
- Use applicationContext instead of activity context when appropriate.
- Follow garbage collection best practices.
- Keep an eye on bitmap memory usage and recycle resources when done.
- Handle lifecycle events to avoid memory leaks.
Resource | Management Tip |
---|---|
Context References | Use weak references to avoid memory leaks |
Bitmap Resources | Recycle and reuse when possible |
Background Tasks | Manage lifecycle events to prevent issues |
Network Resources | Optimize request handling |
With your code streamlined for speed and reliability, you can move on to improving app performance through smarter caching and data handling.
sbb-itb-7af2948
Using Advanced Caching and Data Optimization
Speed and responsiveness are non-negotiable for mobile apps. Smart caching and efficient data handling can cut down load times and make your app perform better overall.
Setting Up Local Caching
Local caching helps apps run smoothly, even with spotty internet connections. It minimizes server requests and improves the user experience by storing data closer to the user.
Cache Type | Ideal Use Case | Tools to Use |
---|---|---|
Memory Cache | UI elements, session data | NSCache (iOS), LruCache (Android) |
Disk Cache | Images, videos, large files | Core Data (iOS), Room (Android) |
Database Cache | User data, configurations | SQLite, Realm |
To get the most out of caching, focus on setting appropriate cache size limits, creating invalidation rules, compressing files, and regularly monitoring performance.
Utilizing Content Delivery Networks (CDNs)
Companies like Netflix rely on CDNs to deliver content quickly, even to millions of users. Techniques like enabling GZIP or Brotli compression, using edge computing to minimize latency, and setting cache headers correctly can significantly enhance content delivery.
Optimizing Database Queries
Efficient database queries are essential as apps grow more complex. Pinterest, for example, cut load times by 30% and boosted user engagement by 25% through database optimizations and caching [2].
Technique | Benefit |
---|---|
Query Indexing | Speeds up data retrieval |
Data Normalization | Reduces redundancy |
Connection Pooling | Cuts down overhead |
Query Caching | Shortens response times |
For apps managing large datasets, tools like Redis or Memcached can help. These in-memory caching solutions store frequently accessed data, allowing for faster retrieval without overloading the database.
Once caching and data optimization are in place, you can turn your attention to improving network requests for even better performance.
Improving Network Requests for Better Connectivity
Optimizing network requests is essential for ensuring apps are responsive and reliable, even in areas with poor connectivity. It plays a key role in improving overall app performance.
Reducing and Streamlining API Calls
Cutting down on unnecessary API calls can make your app more efficient. Combining related requests into a single call helps reduce overhead and save battery life, which is especially important for real-time applications.
Request Type | Strategy | Benefit |
---|---|---|
User-initiated | Limit request frequency | Saves battery power |
Background sync | Group requests | Reduces network usage |
Real-time updates | Use event-driven methods (e.g., FCM) | Fewer active connections |
Using Data Serialization and Compression
Switching to Protocol Buffers instead of JSON can shrink payload sizes, making data transfer quicker. GZIP compression is another way to cut down on data volumes, especially for text-heavy responses.
For example, Instagram used advanced compression techniques for image transfers, cutting data transfer sizes by 25% without sacrificing image quality.
Managing Network Issues Effectively
Strong error-handling mechanisms are critical for maintaining reliability. Here are some effective strategies:
Strategy | Purpose | How to Implement |
---|---|---|
Exponential backoff | Avoid server overload | Increase retry intervals gradually |
Background processing | Keep the app responsive | Use tools like WorkManager for essential tasks |
A queue system can store failed requests and retry them when the connection improves, ensuring a seamless user experience. Additionally, enabling HTTP/2 can boost performance by allowing multiple requests over a single connection.
Platforms like Firebase Performance Monitoring offer tools to track these optimizations and pinpoint bottlenecks in real-time [5].
Once these network optimizations are in place, rigorous testing and monitoring are necessary to confirm their effectiveness in real-world conditions.
Using Performance Testing and Monitoring Tools
Today’s tools offer detailed insights into app behavior, helping developers spot and address issues before they affect users.
Performance Testing and Debugging Tools
Automated performance testing tools, like Firebase Test Lab, simulate user interactions across various devices and networks to detect performance bottlenecks early.
Testing Aspect | Tool | Purpose |
---|---|---|
UI Response | Appium | Automated testing across platforms |
Load Testing | Firebase Test Lab | Testing on real devices and configurations |
Network Simulation | Charles Proxy | Simulates various network conditions |
Profiling tools take it a step further by analyzing app resource usage. For instance, Android Studio Profiler and Xcode Instruments provide real-time data on CPU usage, memory allocation, and network request delays.
"Slow apps lead to poor reviews and lost business, making performance optimization essential." – Google Developers, Android Performance Best Practices [1]
Performance Metric | Target Range & Impact |
---|---|
Resource Usage | CPU < 15%, Memory < 80% RAM; Prevents crashes and keeps UI smooth |
Network Latency | Under 300ms; Ensures fast response times |
Implementing Continuous Monitoring and Updates
After launch, consistent monitoring is crucial to maintain app performance. Tools like Google Analytics and Firebase Performance Monitoring deliver real-time data on app behavior, tracking KPIs and spotting new issues as they arise.
Key monitoring practices include:
Practice | Purpose | Tool |
---|---|---|
Real-time Metrics | Monitor current performance | Firebase Performance Monitoring |
Crash Analytics | Detect and resolve stability issues | Crashlytics |
User Flow Analysis | Analyze critical user paths | Google Analytics |
These tools complement earlier strategies, such as caching and optimizing network usage, creating a well-rounded approach to app performance. By using these resources effectively, developers can keep their apps fast, stable, and enjoyable for users long after release.
Conclusion: Building a High-Performing Mobile App
Key Strategies for Success
To create apps that meet user demands for speed and reliability, certain strategies are essential. A well-thought-out architecture and efficient coding practices form the core of app performance. Studies show that 70% of users abandon apps with slow load times [1].
Performance Area | Implementation Tactics |
---|---|
Architecture | Use native features, modular design |
Code Efficiency | Apply asynchronous programming, manage memory effectively |
Data Handling | Enable local caching, use CDNs |
Network Setup | Streamline requests, compress data |
Expertise in Custom App Development Matters
As highlighted earlier, using native features and modular design ensures scalability. Success comes from blending technical accuracy with continuous fine-tuning. This can be achieved through:
- Regular performance monitoring
- Testing on actual devices
- Ongoing improvements based on performance insights